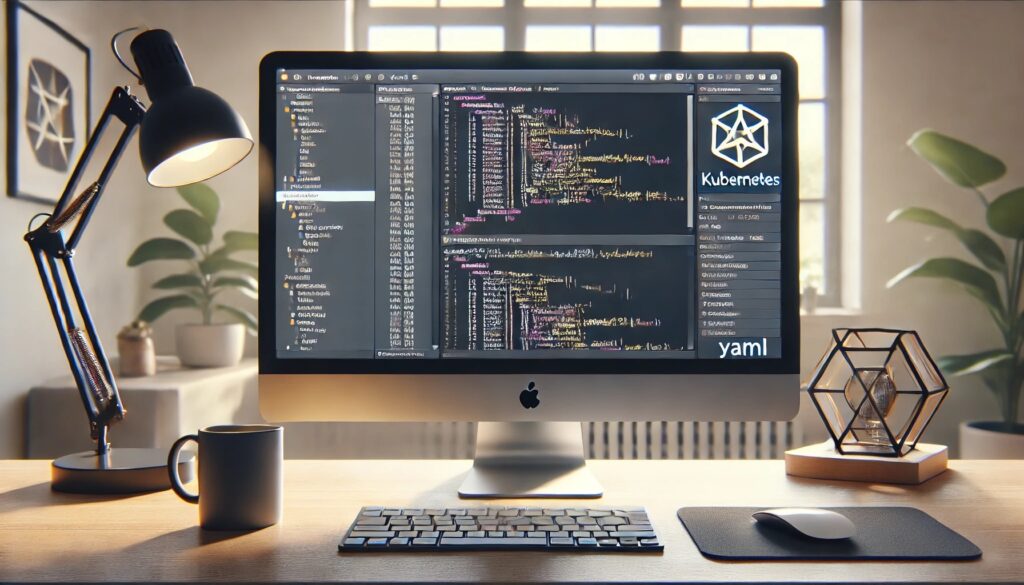
When you first start using Kubernetes, Pods might seem straightforward. Initially, they look like simple containers grouped, right? But hidden beneath this simplicity are powerful techniques that can elevate your Kubernetes deployments from merely functional to exceptionally robust, efficient, and secure. Let’s explore these advanced Kubernetes Pod concepts and empower DevOps engineers, Site Reliability Engineers (SREs), and curious developers to build better, stronger, and smarter systems.
Multi-Container Pods, a Closer Look
Beginners typically deploy Pods containing just one container. But Kubernetes offers more: you can bundle several containers within a single Pod, letting them efficiently share resources like network and storage.
Sidecar pattern in Action
Imagine giving your application a helpful partner, that’s what a sidecar container does. It’s like having a dependable assistant who quietly manages important details behind the scenes, allowing you to focus on your primary tasks without distraction. A sidecar container handles routine but essential responsibilities such as logging, monitoring, or data synchronization, tasks your main application shouldn’t need to worry about directly. For instance, while your main app engages users, responds to requests, and processes transactions, the sidecar can quietly collect logs and forward them efficiently to a logging system. This clever separation of concerns simplifies development and enhances reliability by isolating additional functionality neatly alongside your main application.
containers:
- name: primary-app
image: my-cool-app
- name: log-sidecar
image: logging-agent
Adapter and ambassador patterns explained
Adapters are essentially translators, they take your application’s outputs and reshape them into forms that other external systems can easily understand. Think of them as diplomats who speak the language of multiple systems, bridging communication gaps effortlessly. Ambassadors, on the other hand, serve as intermediaries or dedicated representatives, handling external interactions on behalf of your main container. Imagine your application needing frequent access to an external API; the ambassador container could manage local caching and simplify interactions, reducing latency and speeding up response times dramatically. Both adapters and ambassadors cleverly streamline integration and improve overall system efficiency by clearly defining responsibilities and interactions.
Init containers, setting the stage
Before your Pod kicks into gear and starts its primary job, there’s usually a bit of groundwork to lay first. Just as you might check your toolbox and gather your materials before starting a project, init containers take care of essential setup tasks for your Pods. These handy containers run before the main application container and handle critical chores such as verifying database connections, downloading necessary resources, setting up configuration files, or tweaking file permissions to ensure everything is in the right state. By using init containers, you’re ensuring that when your application finally says, “Ready to go!”, it is ready, avoiding potential hiccups and smoothing out your application’s startup process.
initContainers:
- name: initial-setup
image: alpine
command: ["sh", "-c", "echo Environment setup complete!"]
Strengthening Pod stability with disruption budgets
Pods aren’t permanent; they can be disrupted by routine maintenance or unexpected failures. Pod Disruption Budgets (PDBs) keep services running smoothly by ensuring a minimum number of Pods remain active, even during disruptions.
apiVersion: policy/v1
kind: PodDisruptionBudget
metadata:
name: stable-app
spec:
minAvailable: 2
selector:
matchLabels:
app: stable-app
This setup ensures Kubernetes maintains at least two active Pods at all times.
Scheduling mastery with Pod affinity and anti-affinity
Affinity and anti-affinity rules help Kubernetes make smart decisions about Pod placement, almost as if the Pods themselves have preferences about where they want to live. Think of affinity rules as Pods that prefer to hang out together because they benefit from proximity, like friends working better in the same office. For instance, clustering database Pods together helps reduce latency, ensuring faster communication. On the other hand, anti-affinity rules act more like Pods that prefer their own space, spreading frontend Pods across multiple nodes to ensure that if one node experiences trouble, others continue operating smoothly. By mastering these strategies, you enable Kubernetes to optimize your application’s performance and resilience in a thoughtful, almost intuitive manner.
Affinity example (Grouping Together):
affinity:
podAffinity:
requiredDuringSchedulingIgnoredDuringExecution:
- labelSelector:
matchExpressions:
- key: role
operator: In
values:
- database
topologyKey: "kubernetes.io/hostname"
Anti-Affinity example (Spreading Apart):
affinity:
podAntiAffinity:
requiredDuringSchedulingIgnoredDuringExecution:
- labelSelector:
matchExpressions:
- key: role
operator: In
values:
- webserver
topologyKey: "kubernetes.io/hostname"
Pod health checks. Readiness, Liveness, and Startup Probes
Kubernetes regularly checks the health of your Pods through:
- Readiness Probes: Confirm your Pod is ready to handle traffic.
- Liveness Probes: Continuously check Pod responsiveness and restart if necessary.
- Startup Probes: Give Pods ample startup time before running other probes.
startupProbe:
httpGet:
path: /status
port: 8080
initialDelaySeconds: 30
periodSeconds: 10
Resource management with requests and limits
Pods need resources like CPU and memory, much like how you need food and energy to stay productive throughout the day. But just as you shouldn’t overeat or exhaust yourself, Pods should also be careful with resource usage. Kubernetes provides an elegant solution to this challenge by letting you politely request the resources your Pod requires and firmly setting limits to prevent excessive consumption. This thoughtful management ensures every Pod gets its fair share, maintaining harmony in the shared environment, and helping prevent resource-starvation issues that could slow down or disrupt the entire system.
resources:
requests:
cpu: "250m"
memory: "256Mi"
limits:
cpu: "750m"
memory: "512Mi"
Precise Pod scheduling with taints and tolerations
In Kubernetes, nodes sometimes have specific conditions or labels called “taints.” Think of these taints as signs on the doors of rooms saying, “Only enter if you need what’s inside.” Pods respond to these taints by using something called “tolerations,” essentially a way for Pods to say, “Yes, I recognize the conditions of this node, and I’m fine with them.” This clever mechanism ensures that Pods are selectively scheduled onto nodes best suited for their specific needs, optimizing resources and performance in your Kubernetes environment.
tolerations:
- key: "gpu-enabled"
operator: "Equal"
value: "true"
effect: "NoSchedule"
Ephemeral vs Persistent storage
Ephemeral storage is like scribbling a quick note on a chalkboard, useful for temporary reminders or short-term calculations, but easily erased. When Pods restart, everything stored in ephemeral storage vanishes, making it ideal for temporary data that you won’t miss. Persistent storage, however, is akin to carefully writing down important notes in your notebook, where they’re preserved safely even after you close it. This type of storage maintains its contents across Pod restarts, making it perfect for storing critical, long-term data that your application depends on for continued operation.
Temporary Storage:
volumes:
- name: ephemeral-data
emptyDir: {}
Persistent Storage:
volumes:
- name: permanent-data
persistentVolumeClaim:
claimName: data-pvc
Efficient autoscaling ⏩ Horizontal and Vertical
Horizontal scaling is like having extra hands on deck precisely when you need them. If your application suddenly faces increased traffic, imagine a store suddenly swarming with customers, you quickly bring in additional help by spinning up more Pods. Conversely, when things slow down, you gracefully scale back to conserve resources. Vertical scaling, however, is more about fine-tuning the capabilities of each Pod individually. Think of it as providing a worker with precisely the right tools and workspace they need to perform their job efficiently. Kubernetes dynamically adjusts the resources allocated to each Pod, ensuring they always have the perfect amount of CPU and memory for their workload, no more and no less. These strategies together keep your applications agile, responsive, and resource-efficient.
Horizontal Scaling:
apiVersion: autoscaling/v2
kind: HorizontalPodAutoscaler
metadata:
name: mi-aplicacion-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: mi-aplicacion-deployment
minReplicas: 3
maxReplicas: 10
metrics:
- type: Resource
resource:
name: cpu
target:
type: Utilization
averageUtilization: 75
Vertical Scaling:
apiVersion: autoscaling.k8s.io/v1
kind: VerticalPodAutoscaler
metadata:
name: my-app-vpa
spec:
targetRef:
apiVersion: "apps/v1"
kind: Deployment
name: my-app-deployment
updatePolicy:
updateMode: "Auto" # "Auto", "Off", "Initial"
resourcePolicy:
containerPolicies:
- containerName: '*'
minAllowed:
cpu: 100m
memory: 256Mi
maxAllowed:
cpu: 1
memory: 1Gi
Enhancing Pod Security with Network Policies
Network policies act like traffic controllers for your Pods, deciding who talks to whom and ensuring unwanted visitors stay away. Imagine hosting an exclusive gathering, only guests are allowed in. Similarly, network policies permit Pods to communicate strictly according to defined rules, enhancing security significantly. For instance, you might allow only your frontend Pods to interact directly with backend Pods, preventing potential intruders from sneaking into sensitive areas. This strategic control keeps your application’s internal communications safe, orderly, and efficient.
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: frontend-backend-policy
spec:
podSelector:
matchLabels:
app: backend
ingress:
- from:
- podSelector:
matchLabels:
app: frontend
Empowering your Kubernetes journey
Now imagine you’re standing in a vast workshop, tools scattered around you. At first glance, a Pod seems like a simple wooden box, unassuming, almost ordinary. But open it up, and inside you’ll find gears, springs, and levers arranged with precision. Each component has a purpose, and when you learn to tweak them just right, that humble box transforms into something extraordinary: a clock that keeps perfect time, a music box that hums symphonies, or even a tiny engine that powers a locomotive.
That’s the magic of mastering Kubernetes Pods. You’re not just deploying containers; you’re orchestrating tiny ecosystems. Think of the sidecar pattern as adding a loyal assistant who whispers, “Don’t worry about the logs, I’ll handle them. You focus on the code.” Or picture affinity rules as matchmakers, nudging Pods to cluster together like old friends at a dinner party, while anti-affinity rules act likewise parents, saying, “Spread out, kids, no crowding the kitchen!”
And what about those init containers? They’re the stagehands of your Pod’s theater. Before the spotlight hits your main app, these unsung heroes sweep the floor, adjust the curtains, and test the microphones. No fanfare, just quiet preparation. Without them, the show might start with a screeching feedback loop or a missing prop.
But here’s the real thrill: Kubernetes isn’t a rigid rulebook. It’s a playground. When you define a Pod Disruption Budget, you’re not just setting guardrails, you’re teaching your cluster to say, “I’ll bend, but I won’t break.” When you tweak resource limits, you’re not rationing CPU and memory; you’re teaching your apps to dance gracefully, even when the music speeds up.
And let’s not forget security. With Network Policies, you’re not just building walls, you’re designing secret handshakes. “Psst, frontend, you can talk to the backend, but no one else gets the password.” It’s like hosting a masquerade ball where every guest is both mysterious and meticulously vetted.
So, what’s the takeaway? Kubernetes Pods aren’t just YAML files or abstract concepts. They’re living, breathing collaborators. The more you experiment, tinkering with probes, laughing at the quirks of taints and tolerations, or marveling at how ephemeral storage vanishes like chalk drawings in the rain, the more you’ll see patterns emerge. Patterns that whisper, “This is how systems thrive.”
Will there be missteps? Of course! Maybe a misconfigured probe or a Pod that clings to a node like a stubborn barnacle. But that’s the joy of it. Every hiccup is a puzzle and every solution? A tiny epiphany. So go ahead, grab those Pods, twist them, prod them, and watch as your deployments evolve from “it works” to “it sings.” The journey isn’t about reaching perfection. It’s about discovering how much aliveness you can infuse into those lines of YAML. And trust me, the orchestra you’ll conduct? It’s worth every note.