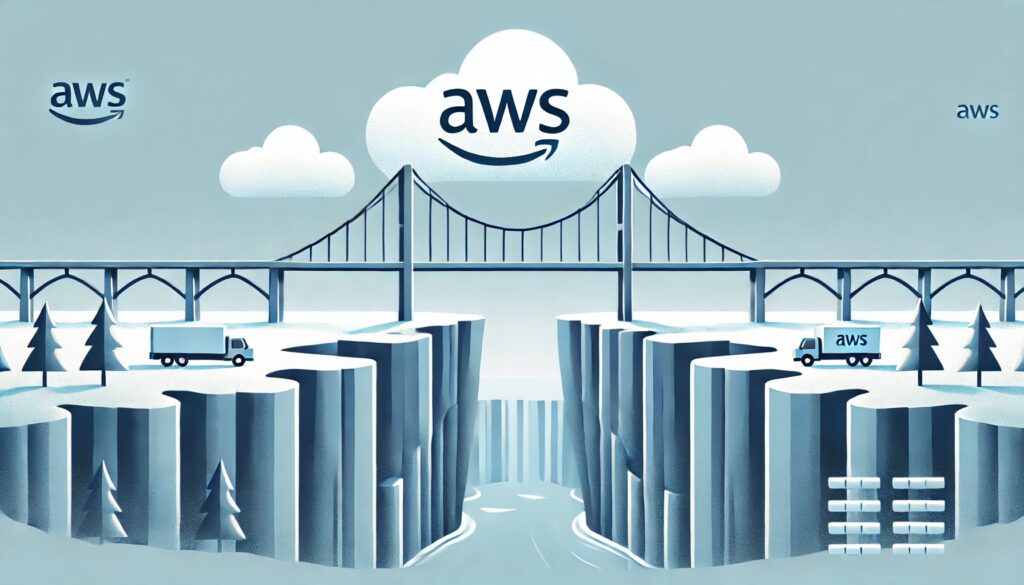
Let’s talk about something really important, even if it’s not always the most glamorous topic: keeping your AWS-based applications running, no matter what. We’re going to explore the world of High Availability (HA) and Disaster Recovery (DR). Think of it as building a castle strong enough to withstand a dragon attack, or, you know, a server outage..
Why all the fuss about Disaster Recovery?
Businesses run on applications. These are the engines that power everything from online shopping to, well, pretty much anything digital. If those engines sputter and die, bad things happen. Money gets lost. Customers get frustrated. Reputations get tarnished. High Availability and Disaster Recovery are all about making sure those engines keep running, even when things go wrong. It’s about resilience.
Before we jump into solutions, we need to understand two key measurements:
- Recovery Time Objective (RTO): How long can you afford to be down? Minutes? Hours? Days? This is your RTO.
- Recovery Point Objective (RPO): How much data can you afford to lose? The last hour’s worth? The last days? That’s your RPO.
Think of RTO and RPO as your “pain tolerance” levels. A low RTO and RPO mean you need things back up and running fast, with minimal data loss. A higher RTO and RPO mean you can tolerate a bit more downtime and data loss. The correct option will depend on your business needs.
Disaster recovery strategies on AWS, from basic to bulletproof
AWS offers a toolbox of options, from simple backups to fully redundant, multi-region setups. Let’s explore a few common strategies, like choosing the right level of armor for your knight:
- Pilot Light: Imagine keeping the pilot light lit on your stove. It’s not doing much, but it’s ready to ignite the main burner at any moment. In AWS terms, this means having the bare minimum running, maybe a database replica syncing data in another region, and your server configurations saved as templates (AMIs). When disaster strikes, you “turn on the gas”, launch those servers, connect them to the database, and you’re back in business.
- Good for: Cost-conscious applications where you can tolerate a few hours of downtime.
- AWS Services: RDS Multi-AZ (for database replication), Amazon S3 cross-region replication, EC2 AMIs.
- Warm Standby: This is like having a smaller, backup stove already plugged in and warmed up. It’s not as powerful as your main stove, but it can handle the basic cooking while the main one is being repaired. In AWS, you’d have a scaled-down version of your application running in another region. It’s ready to handle traffic, but you might need to scale it up (add more “burners”) to handle the full load.
- Good for: Applications where you need faster recovery than Pilot Light, but you still want to control costs.
- AWS Services: Auto Scaling (to automatically adjust capacity), Amazon EC2, Amazon RDS.
- Active/Active (Multi-Region): This is the “two full kitchens” approach. You have identical setups running in multiple AWS regions simultaneously. If one kitchen goes down, the other one is already cooking, and your customers barely notice a thing. You use AWS Route 53 (think of it as a smart traffic controller) to send users to the closest or healthiest “kitchen.”
- Good for: Mission-critical applications where downtime is simply unacceptable.
- AWS Services: Route 53 (with health checks and failover routing), Amazon EC2, Amazon RDS, DynamoDB global tables.
Picking the right armor, It’s all about trade-offs
There’s no “one-size-fits-all” answer. The best strategy depends on those RTO/RPO targets we talked about, and, of course, your budget.
Here’s a simple way to think about it:
- Tight RTO/RPO, Budget No Object? Active/Active is your champion.
- Need Fast Recovery, But Watching Costs? Warm Standby is a good compromise.
- Can Tolerate Some Downtime, Prioritizing Cost Savings? Pilot Light is your friend.
- Minimum RTO/RPO and Minimum Budget? Backups.
The trick is to be honest about your real needs. Don’t build a fortress if a sturdy wall will do.
A quick glimpse at implementation
Let’s say you’re going with the Pilot Light approach. You could:
- Set up Amazon S3 Cross-Region Replication to copy your important data to another AWS region.
- Create an Amazon Machine Image (AMI) of your application server. This is like a snapshot of your server’s configuration.
- Store that AMI in the backup region.
In a disaster scenario, you’d launch EC2 instances from that AMI, connect them to your replicated data, and point your DNS to the new instances.
Tools like AWS Elastic Disaster Recovery (a managed service) or CloudFormation (for infrastructure-as-code) can automate much of this process, making it less of a headache.
Testing, Testing, 1, 2, 3…
You wouldn’t buy a car without a test drive, right? The same goes for disaster recovery. You must test your plan regularly.
Simulate a failure. Shut down resources in your primary region. See how long it takes to recover. Use AWS CloudWatch metrics to measure your actual RTO and RPO. This is how you find the weak spots before a real disaster hits. It’s like fire drills for your application.
The takeaway, be prepared, not scared
Disaster recovery might seem daunting, but it doesn’t have to be. AWS provides the tools, and with a bit of planning and testing, you can build a resilient architecture that can weather the storm. It’s about peace of mind, knowing that your business can keep running, no matter what. Start small, test often, and build up your defenses over time.