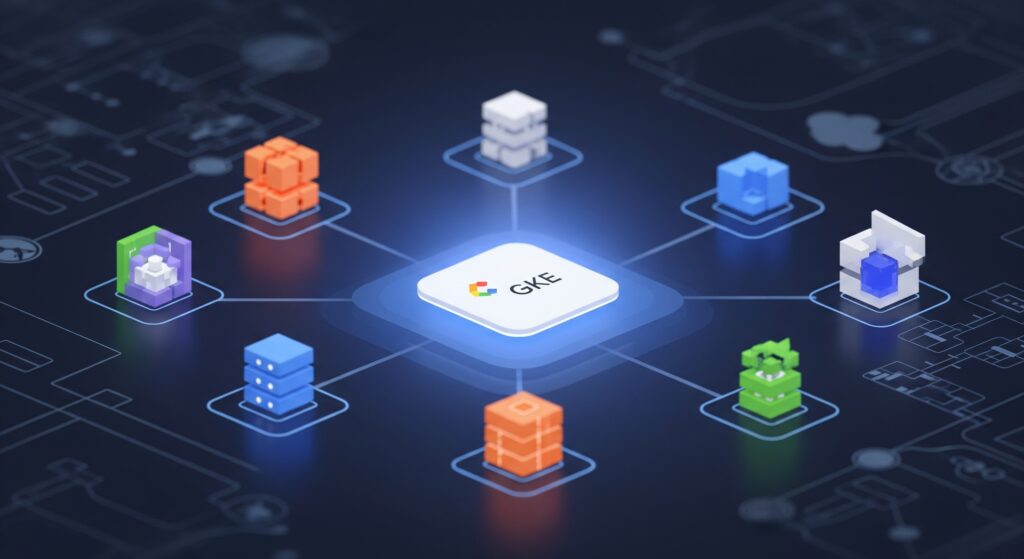
Exploring the world of containerized applications reveals Kubernetes as the essential conductor for its intricate operations. It’s the common language everyone speaks, much like how standard shipping containers revolutionized global trade by fitting onto any ship or truck. Many cloud providers offer their own managed Kubernetes services, but Google Kubernetes Engine (GKE) often takes center stage. It’s not just another Kubernetes offering; its deep roots in Google Cloud, advanced automation, and unique optimizations make it a compelling choice.
Let’s see what sets GKE apart from alternatives like Amazon EKS, Microsoft AKS, and self-managed Kubernetes, and explore why it might be the most robust platform for your cloud-native ambitions.
Google’s inherent Kubernetes expertise
To truly understand GKE’s edge, we need to look at its origins. Google didn’t just adopt Kubernetes; they invented it, evolving it from their internal powerhouse, Borg. Think of it like learning a complex recipe. You could learn from a skilled chef who has mastered it, or you could learn from the very person who created the dish, understanding every nuance and ingredient choice. That’s GKE.
This “creator” status means:
- Direct, Unfiltered Expertise: GKE benefits directly from the insights and ongoing contributions of the engineers who live and breathe Kubernetes.
- Early Access to Innovation: GKE often supports the latest stable Kubernetes features before competitors can. It’s like getting the newest tools straight from the workshop.
- Seamless Google Cloud Synergy: The integration with Google Cloud services like Cloud Logging, Cloud Monitoring, and Anthos is incredibly tight and natural, not an afterthought.
How Others Compare:
While Amazon EKS and Microsoft AKS are capable managed services, they don’t share this native lineage. Self-managed Kubernetes, whether on-premises or set up with tools like kops, places the full burden of upgrades, maintenance, and deep expertise squarely on your shoulders.
The simplicity of Autopilot fully managed Kubernetes
GKE offers a game-changing operational model called Autopilot, alongside its Standard mode (which is more akin to EKS/AKS where you manage node pools). Autopilot is like hiring an expert event planning team that also handles all the setup, catering, and cleanup for your party, leaving you to simply enjoy hosting. It offers a truly serverless Kubernetes experience.
Key benefits of Autopilot:
- Zero Node Management: Google takes care of node provisioning, scaling, and all underlying infrastructure concerns. You focus on your applications, not the plumbing.
- Optimized Cost Efficiency: You pay for the resources your pods actually consume, not for idle nodes. It’s like only paying for the electricity your appliances use, not a flat fee for being connected to the grid.
- Built-in Enhanced Security: Security best practices are automatically applied and managed by Google, hardening your clusters by default.
How others compare:
EKS and AKS require you to actively manage and scale your node pools. Self-managed clusters demand significant, ongoing operational efforts to keep everything running smoothly and securely.
Unified multi-cluster and multi-cloud operations with Anthos
In an increasingly distributed world, managing applications across different environments can feel like juggling too many balls. GKE’s integration with Anthos, Google’s hybrid and multi-cloud platform, acts as a master control panel.
Anthos allows for:
- Centralized command: Manage GKE clusters alongside those on other clouds like EKS and AKS, and even your on-premises deployments, all from a single viewpoint. It’s like having one universal remote for all your different entertainment systems.
- Consistent policies everywhere: Apply uniform configurations and security policies across all your environments using Anthos Config Management, ensuring consistency no matter where your workloads run.
- True workload portability: Design for flexibility and avoid vendor lock-in, moving applications where they make the most sense.
How Others Compare:
EKS and AKS generally lack such comprehensive, native multi-cloud management tools. Self-managed Kubernetes often requires integrating third-party solutions like Rancher to achieve similar multi-cluster oversight, adding complexity.
Sophisticated networking and security foundations
GKE comes packed with unique networking and security features that are deeply woven into the platform.
Networking highlights:
- Global load balancing power: Native integration with Google’s global load balancer means faster, more scalable, and more resilient traffic management than many traditional setups.
- Automated certificate management: Google-managed Certificate Authority simplifies securing your services.
- Dataplane V2 advantage: This Cilium-based networking stack provides enhanced security, finer-grained policy enforcement, and better observability. Think of it as upgrading your building’s basic security camera system to one with AI-powered threat detection and detailed access logs.
Security fortifications:
- Workload identity clarity: This is a more secure way to grant Kubernetes service accounts access to Google Cloud resources. Instead of managing static, exportable service account keys (like having physical keys that can be lost or copied), each workload gets a verifiable, short-lived identity, much like a temporary, auto-expiring digital pass.
- Binary authorization assurance: Enforce policies that only allow trusted, signed container images to be deployed.
- Shielded GKE nodes protection: These nodes benefit from secure boot, vTPM, and integrity monitoring, offering a hardened foundation for your workloads.
How Others Compare:
While EKS and AKS leverage AWS and Azure security tools respectively, achieving the same level of integration, Kubernetes-native security often requires more manual configuration and piecing together different services. Self-managed clusters place the entire burden of security hardening and ongoing vigilance on your team.
Smart cost efficiency and pricing structure
GKE’s pricing model is competitive, and Autopilot, in particular, can lead to significant savings.
- No control plane fees for Autopilot: Unlike EKS, which charges an hourly fee per cluster control plane, GKE Autopilot clusters don’t have this charge. Standard GKE clusters have one free zonal cluster per billing account, with a small hourly fee for regional clusters or additional zonal ones.
- Sustained use discounts: Automatic discounts are applied for workloads that run for extended periods.
- Cost-Saving VM options: Support for Preemptible VMs and Spot VMs allows for substantial cost reductions for fault-tolerant or batch workloads.
How Others Compare:
EKS incurs control plane costs on top of node costs. AKS offers a free control plane but may not match GKE’s automation depth, potentially leading to other operational costs.
Optimized for AI ML and Big Data workloads
For teams working with Artificial Intelligence, Machine Learning, or Big Data, GKE offers a highly optimized environment.
- Seamless GPU and TPU access: Effortless provisioning and utilization of GPUs and Google’s powerful TPUs.
- Kubeflow integration: Streamlines the deployment and management of ML pipelines.
- Strong BigQuery ML and Vertex AI synergy: Tight compatibility with Google’s leading data analytics and AI platforms.
How Others Compare:
EKS and AKS support GPUs, but native TPU integration is a unique Google Cloud advantage. Self-managed setups require manual configuration and integration of the entire ML stack.
Why GKE stands out
Choosing the right Kubernetes platform is crucial. While all managed services aim to simplify Kubernetes operations, GKE offers a unique blend of heritage, innovation, and deep integration.
GKE emerges as a firm contender if you prioritize:
- A truly hands-off, serverless-like Kubernetes experience with Autopilot.
- The benefits of Google’s foundational Kubernetes expertise and rapid feature adoption.
- Seamless hybrid and multi-cloud capabilities through Anthos.
- Advanced, built-in security and networking designed for modern applications.
If your workloads involve AI/ML, and big data analytics, or you’re deeply invested in the Google Cloud ecosystem, GKE provides an exceptionally integrated and powerful experience. It’s about choosing a platform that not only manages Kubernetes but elevates what you can achieve with it.