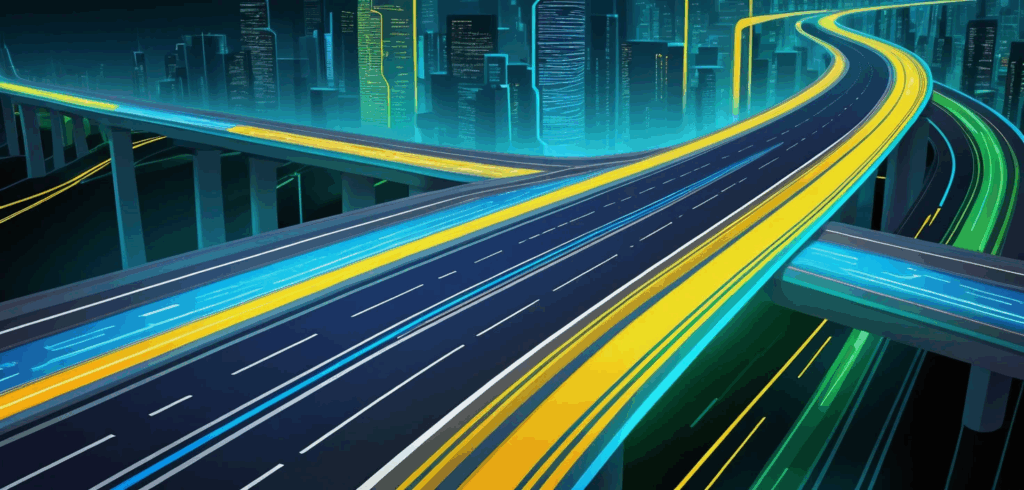
A sluggish CI/CD pipeline is more than an inconvenience, it’s like standing in a seemingly endless queue at your favorite coffee shop every single morning. Each delay wastes valuable time, steadily draining motivation and productivity.
Let’s share some practical, effective strategies that have significantly reduced pipeline delays in my projects, creating smoother, faster, and more dependable workflows.
Identifying common pipeline bottlenecks
Before exploring solutions, let’s identify typical pipeline issues:
- Inefficient or overly complex scripts
- Tasks executed sequentially rather than in parallel
- Redundant deployment steps
- Unoptimized Docker builds
- Fresh installations of dependencies for every build
By carefully analyzing logs, reviewing performance metrics, and manually timing each stage, it became clear where improvements could be made.
Reviewing the Initial Pipeline Setup
Initially, the pipeline consisted of:
- Unit testing
- Integration testing
- Application building
- Docker image creation and deployment
Testing stages were the biggest consumers of time, followed by Docker image builds and overly intricate deployment scripts.
Introducing parallel execution
Allowing independent tasks to run simultaneously rather than sequentially greatly reduced waiting times:
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Install Dependencies
run: npm ci
- name: Run Unit Tests
run: npm run test:unit
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Install Dependencies
run: npm ci
- name: Build Application
run: npm run build
This adjustment improved responsiveness, significantly reducing idle periods.
Utilizing caching to prevent redundancy
Constantly reinstalling dependencies was like repeatedly buying groceries without checking the fridge first. Implementing caching for Node modules substantially reduced these repetitive installations:
- name: Cache Node Modules
uses: actions/cache@v3
with:
path: ~/.npm
key: ${{ runner.os }}-npm-${{ hashFiles('**/package-lock.json') }}
restore-keys: |
${{ runner.os }}-npm-
Streamlining tests based on changes
Running every test for each commit was unnecessarily exhaustive. Using Jest’s –changedSince flag, tests became focused on recent modifications:
npx jest --changedSince=main
This targeted approach optimized testing time without compromising test coverage.
Optimizing Docker builds with Multi-Stage techniques
Docker image creation was initially a major bottleneck. Switching to multi-stage Docker builds simplified the process and resulted in smaller, quicker images:
# Build stage
FROM node:18-alpine as builder
WORKDIR /app
COPY package*.json ./
RUN npm ci
COPY . .
RUN npm run build
# Production stage
FROM nginx:alpine
COPY --from=builder /app/dist /usr/share/nginx/html
The outcome was faster, more efficient builds.
Leveraging scalable Cloud-Based runners
Moving to cloud-hosted runners such as AWS spot instances provided greater speed and scalability. This method, especially beneficial for critical branches, effectively balanced performance and cost.
Key lessons
- Native caching options vary between CI platforms, so external tools might be required.
- Reducing idle waiting is often more impactful than shortening individual task durations.
- Parallel tasks are beneficial but require careful management to avoid overwhelming subsequent processes.
Results achieved
- Significantly reduced pipeline execution time
- Accelerated testing cycles
- Docker builds ceased to be a pipeline bottleneck
Additionally, the overall developer experience improved considerably. Faster feedback cycles, smoother merges, and less stressful releases were immediate benefits.
Recommended best practices
- Run tasks concurrently wherever practical
- Effectively cache dependencies
- Focus tests on relevant code changes
- Employ multi-stage Docker builds for efficiency
- Relocate intensive tasks to scalable infrastructure
Concluding thoughts
Your CI/CD pipeline deserves attention, perhaps as much as your coffee machine. After all, neglect it and you’ll soon find yourself facing cranky developers and sluggish software. Give your pipeline the tune-up it deserves, remove those pesky friction points, and you might just find your developers smiling (yes, smiling!) on deployment days. Remember, your pipeline isn’t just scripts and containers, it’s your project’s slightly neurotic, always evolving, very vital circulatory system. Treat it well, and it’ll keep your software sprinting like an Olympic athlete, rather than limping like a sleep-deprived zombie.