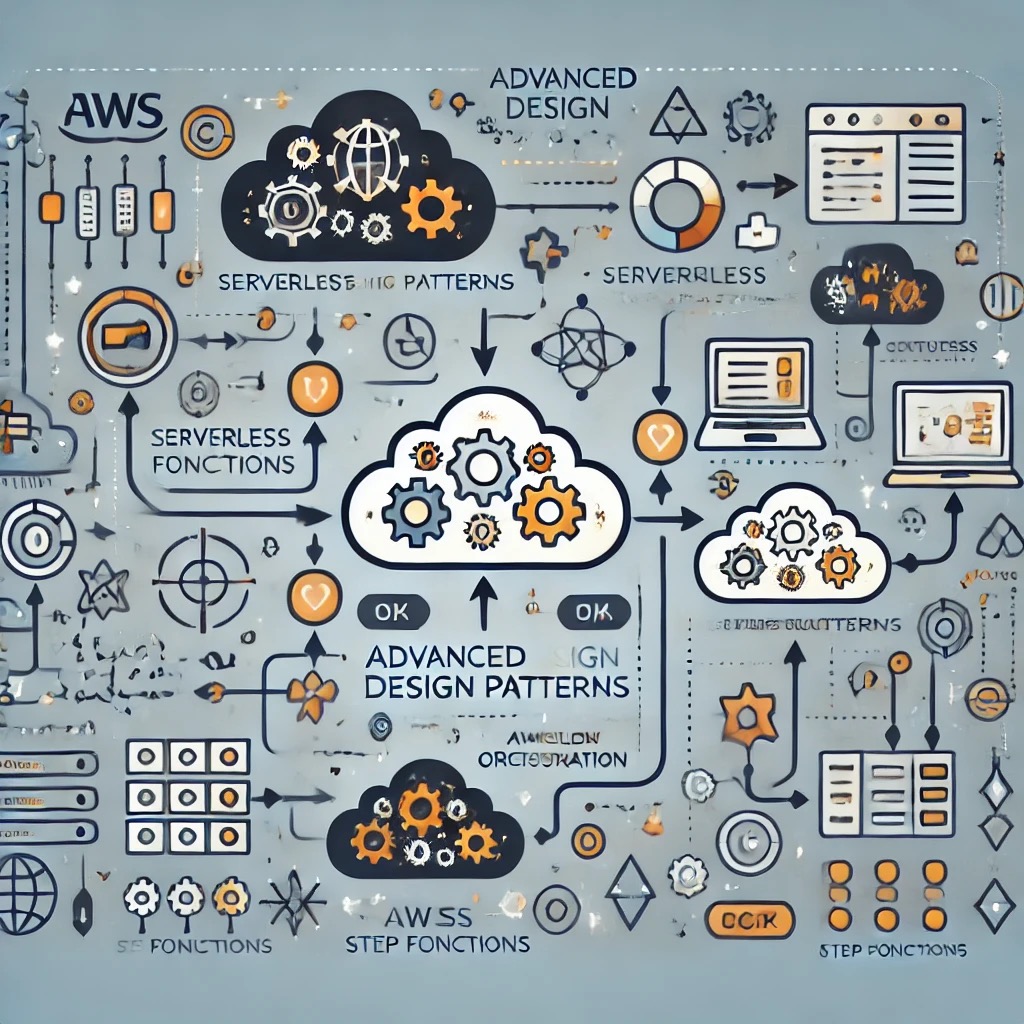
Suppose you’re leading a dance where each partner is a different cloud service, each moving precisely in time. That’s what AWS Step Functions lets you do! AWS Step Functions helps you orchestrate your serverless applications as if you had a magic wand, ensuring each part plays its tune at the right moment. And just like a conductor uses musical patterns, we have design patterns in Step Functions that make this orchestration smooth and efficient.
In this article, we’re embarking on an exciting journey to explore these patterns. We’ll break down complex ideas into simple terms, so even if you’re new to Step Functions, you’ll feel confident and ready to apply these patterns by the end of this read.
Here’s what we’ll cover:
- A quick recap of what AWS Step Functions is all about.
- Why design patterns are like secret recipes for successful workflows.
- How to use these patterns to build powerful and reliable serverless applications.
Understanding the basics
Before diving into the patterns, let’s ensure we’re all on the same page. Think of a state machine in Step Functions as a flowchart. It has different “states” (like boxes in your flowchart) that represent the steps in your workflow. These states are connected by arrows, showing the order in which things happen.
Pattern 1: The “Waiter” Pattern (Wait-for-Callback with Task Tokens)
Imagine you’re at a restaurant. You order your food, and the waiter gives you a number. That number is like a task token in Step Functions. You don’t just stand at the counter staring at the kitchen, right? You relax and wait for your number to be called.
That’s similar to the Wait-for-Callback pattern. You have a task (like ordering food) that takes a while. Instead of constantly checking if it’s done, you give it a token (like your order number) and do other things. When the task is finished, it uses the token to call you back and say, “Hey, your order is ready!”
Why is this useful?
- It lets your workflow do other things while waiting for a long task.
- It’s perfect for tasks that involve human interaction or external services.
How does it work?
- You start a task and give it a token.
- The task does its thing (maybe it’s waiting for a user to approve something).
- Once done, the task uses the token to signal completion.
- Your workflow continues with the next step.
// Pattern 1: Wait-for-Callback with Task Tokens
{
"StartAt": "WaitForCallback",
"States": {
"WaitForCallback": {
"Type": "Task",
"Resource": "arn:aws:states:::lambda:invoke.waitForTaskToken",
"Parameters": {
"FunctionName": "MyCallbackFunction",
"Payload": {
"TaskToken.$": "$$.Task.Token",
"Input.$": "$.input"
}
},
"Next": "ProcessResult",
"TimeoutSeconds": 3600
},
"ProcessResult": {
"Type": "Task",
"Resource": "arn:aws:states:::lambda:invoke",
"Parameters": {
"FunctionName": "ProcessResultFunction",
"Payload.$": "$"
},
"End": true
}
}
}
Things to keep in mind:
- Make sure you handle errors gracefully, like what happens if the waiter forgets your order?
- Set timeouts so your workflow doesn’t wait forever.
- Keep your tokens safe, just like you wouldn’t want someone else to take your food!
Pattern 2: The “Multitasking” Pattern (Parallel processing with Map States)
Ever wished you could do many things at once? Like washing dishes, cooking, and listening to music simultaneously? That’s what Map States let you do in Step Functions. Imagine you have a basket of apples to peel. Instead of peeling them one by one, you can use a Map State to peel many apples at the same time. Each apple gets its peeling process, and they all happen in parallel.
Why is this awesome?
- It speeds up your workflow by doing many things concurrently.
- It’s great for tasks that can be broken down into independent chunks.
How to use it:
- You have a bunch of items (like our apples).
- The Map State creates a separate path for each item.
- Each path does the same steps but on a different item.
- Once all paths are done, the workflow continues.
// Pattern 2: Map State for Parallel Processing
{
"StartAt": "ProcessImages",
"States": {
"ProcessImages": {
"Type": "Map",
"ItemsPath": "$.images",
"MaxConcurrency": 5,
"Iterator": {
"StartAt": "ProcessSingleImage",
"States": {
"ProcessSingleImage": {
"Type": "Task",
"Resource": "arn:aws:states:::lambda:invoke",
"Parameters": {
"FunctionName": "ImageProcessorFunction",
"Payload.$": "$"
},
"End": true
}
}
},
"Next": "AggregateResults"
},
"AggregateResults": {
"Type": "Task",
"Resource": "arn:aws:states:::lambda:invoke",
"Parameters": {
"FunctionName": "AggregateFunction",
"Payload.$": "$"
},
"End": true
}
}
}
Things to watch out for:
- Don’t overload your system by processing too many things at once.
- Keep an eye on costs, as parallel processing can use more resources.
Pattern 3: The “Try-Again” Pattern (Error handling with Retry Policies)
We all make mistakes, right? Sometimes things go wrong, even in our workflows. But that’s okay. The “Try-Again” pattern helps us deal with these hiccups.
Imagine you’re trying to open a door, but it’s stuck. You wouldn’t just give up after one try, would you? You might try again a few times, maybe with a little more force.
Retry Policies are like that. If a step in your workflow fails, it can automatically try again a few times before giving up.
Why is this important?
- It makes your workflows more resilient to temporary glitches.
- It helps you handle unexpected errors gracefully.
How to set it up:
- You define a Retry Policy for a specific step.
- If that step fails, it automatically retries.
- You can customize how many times it retries and how long it waits between tries.
// Pattern 3: Retry Policy Example
{
"StartAt": "CallExternalService",
"States": {
"CallExternalService": {
"Type": "Task",
"Resource": "arn:aws:states:::lambda:invoke",
"Parameters": {
"FunctionName": "ExternalServiceFunction",
"Payload.$": "$"
},
"Retry": [
{
"ErrorEquals": ["ServiceException", "Lambda.ServiceException"],
"IntervalSeconds": 2,
"MaxAttempts": 3,
"BackoffRate": 2.0
},
{
"ErrorEquals": ["States.Timeout"],
"IntervalSeconds": 1,
"MaxAttempts": 2
}
],
"End": true
}
}
}
Real-world examples:
- Maybe a network connection fails temporarily.
- Or a service you’re using is overloaded.
- With Retry Policies, your workflow can handle these situations like a champ!
Putting It All Together
Now that we’ve learned these cool patterns, let’s see how they work together in the real world. Imagine building an image processing pipeline. Think of having a batch of 100 images. You can use the “Multitasking” pattern to process multiple images concurrently, significantly reducing the total time of the pipeline. If one image fails, the “Try-Again” pattern can retry the processing. And if you need to wait for a human to review an image, the “Waiter” pattern comes to the rescue!
Key Takeaways
- Design patterns are like superpowers for your workflows.
- Each pattern solves a specific problem, so choose wisely.
- By combining patterns, you can build incredibly powerful and resilient applications.
In a few words
These patterns are your allies in crafting effective workflows. By understanding and leveraging them, you can transform complex tasks into manageable processes, ensuring that your serverless architectures are not just operational, but optimized and resilient. The real strength of AWS Step Functions lies in its ability to handle the unexpected, coordinate complex tasks, and make your cloud solutions reliable and scalable. Use these design patterns as tools in your problem-solving toolkit, and you’ll find yourself creating workflows that are efficient, reliable, and easy to maintain.